The journey to becoming a developer is not easy, especially if you want to become a JavaScript developer. On your way to success, you’ll discover and use various tools, a ton of frameworks, and even more libraries.
If you decide to acknowledge all possible sources explaining “the best way of doing something,” everything may seem more daunting than it is. I’ve been in your shoes, and while my journey is not yet complete, I wanted to share an excellent set of links and advice that will help you get immersed in the ecosystem.
The most important thing to keep in mind is that you will have to keep up with the language and the community as they grow.
The basics
Learn the basics of JavaScript development before running out and picking up a framework. That includes the JavaScript syntax, the prototype chain, and DOM manipulation. After you are proficient with this, you can pick up jQuery – a library that will help you achieve good browser compatibility and easier consumption and manipulation of the DOM. Keep in mind that jQuery is something you should know and use, but it is not a one-size-fits-all solution.
While investigating and learning, Mozilla Developer Network (MDN) will be your best friend. It is the ultimate resource for all things JavaScript. From now on, you’ll preface every JavaScript-related web search with MDN
.
Frameworks
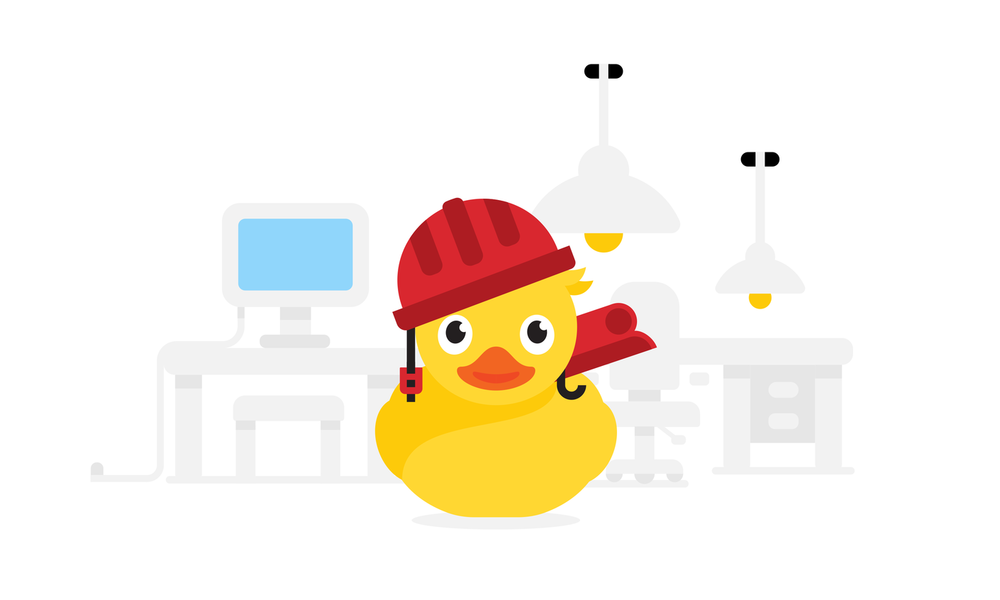
After getting to know the fundamentals, you can start playing around with a framework. There are a lot of frameworks available, but to make things simpler, I’ve hand-picked a few popular ones. Not all projects benefit from using a framework, but a good set of tools, best practices, and paradigms will help you jump-start your JS career.
Angular 1 & 2
You might have also heard about Angular 2. While it shares the name, it’s entirely different from Angular 1. It leverages modern design principles and lessons learned from Angular 1 and other frameworks. It might look complicated at first, but it is certainly worth picking up at some point. You can kick-start your development with a template repository.
Ember
Ember is right behind Angular 2. It provides a full solution: a well-defined architecture that you have to follow, a powerful CLI that hides all the complexity from you, and built-in Handlebars helpers. It also implements common standards so you can focus on your app instead of reinventing the wheel. There’s also a good amount of add-ons. Getting started with Ember should be straightforward due to its excellent guides.
React
React is quite specific since it’s not a framework. It is missing state management (something that stores your data), and you’ll hear its name in conjunction with Redux quite a lot. React is best learned by trying it out. For an easy setup take a look at the create-react-app generator. I’ll also mention our state management library of choice – MobX. It’s much easier to start with compared to Redux, and it will help you understand and implement state management in your app with just a few lines of code.
Backbone
A beginner should also consider Backbone since it provides a full experience which is more bare-bones than others. There are a lot of projects in production that work perfectly with Backbone, so it’s worth picking up and mastering.
Vue.js
I will also mention Vue.js, a framework you’ll be hearing more and more about. You’ll see almost instantly that its components look a lot like React and have a templating language similar to Angular 1.
Many of these frameworks can be seen live with sample source code on a project called Todo MVC. I recommend it as a starting point so you can get a feel of how the projects are structured.
But, as I said before – don’t bother learning all of them. Pick and understand one, and you’ll be able to master all other frameworks with relative ease. A great starting point is the current state of JavaScript frameworks.
Understand your tools and use them
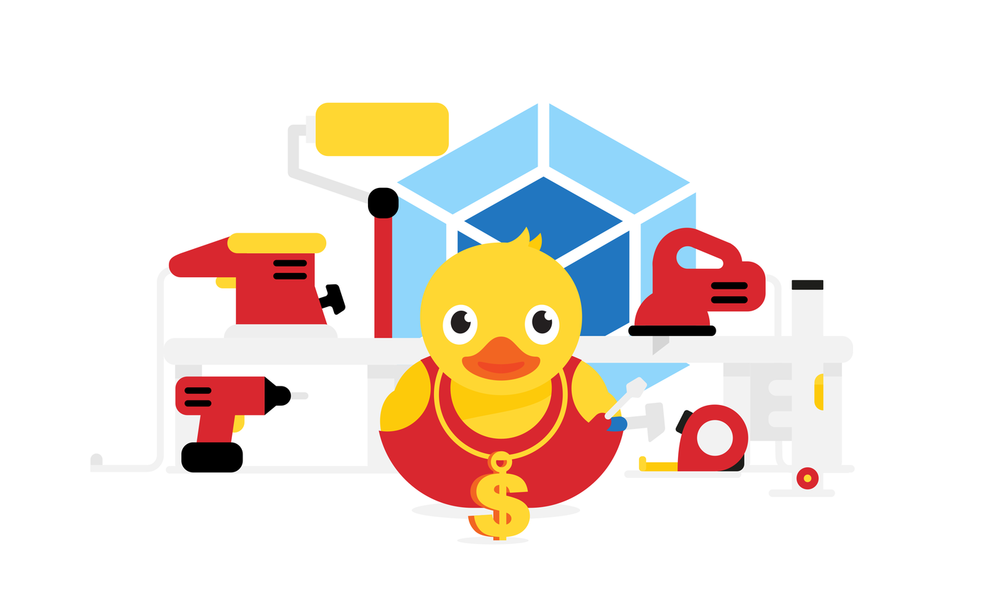
Sooner or later you will need a set of tools in order to amp up your development. They range from basic ones like linting tools (ensuring your code is up to par), up to more complex ones, like build systems that prepare your work of art for deployment. The same principle applies here as well – learn the basics before you fully dive in.
CLI
Editors
If you ever worked with something like Java or C# (ASP.NET), you value your IDE. But with JavaScript, any old plain text editor will work better than a “JavaScript IDE.” What most of them try to accomplish, be it autocompletion or build system integrations, will get in your way because JavaScript is not a statically typed language. That doesn’t mean that you can’t use great editors like Atom, Sublime Text, or Visual Studio Code and empower them with just a few dozen plugins. You can even make everything shake. We use Atom and Visual Studio Code, but my personal recommendation is Visual Studio Code.
Debugging
I can already hear you shouting “you can’t debug without an IDE, and that’s why JavaScript is hard.” My answer is – use Chrome DevTools. Out of every tool available today, the Chrome Developer tools win most of the time. Investing time to learn and master them will pay with interest in future.
Package management
Another tool you’ll hear a lot about is a package manager called NPM – a registry of libraries and frameworks (both for JS and CSS). Advantages of installing your dependencies (e.g. jQuery) via a package manager instead of just adding them to <head>
are speed and having a better control of your dependencies.
Build systems
Last but not least – build systems. You use build systems to optimize your code (e.g. bundle all of the JavaScript files into one), make your code significantly smaller, more optimized, and harder to read (obfuscated). You can use them to copy your assets (fonts, styles, images, etc.) and upload everything to your servers. Each build system takes some time to master, but rest assured you’ll benefit from using one.
Grunt is a popular tool, but it’s becoming outdated, even though you might still find it used in production. Its strengths were that you had a working build system after just a few lines of code. Compared to some other tools, Grunt is slower and not as versatile.
Gulp is similar, but a bit more versatile. Unlike Grunt, this tool will build everything side to side (asynchronously), so it can get amazingly fast real soon. Using Gulp is a safe bet today.
Webpack is the last one I’m going to mention. It is a very powerful and complex tool, but it also happens to be our favorite. The best way of mastering Webpack is following the official documentation. The way it works is probably too complicated for this text and might warrant a post by itself, but to keep things short – it does a lot out of the box. After getting used to its ways, you will get a robust build system for any target.
Picking up your marbles and moving on
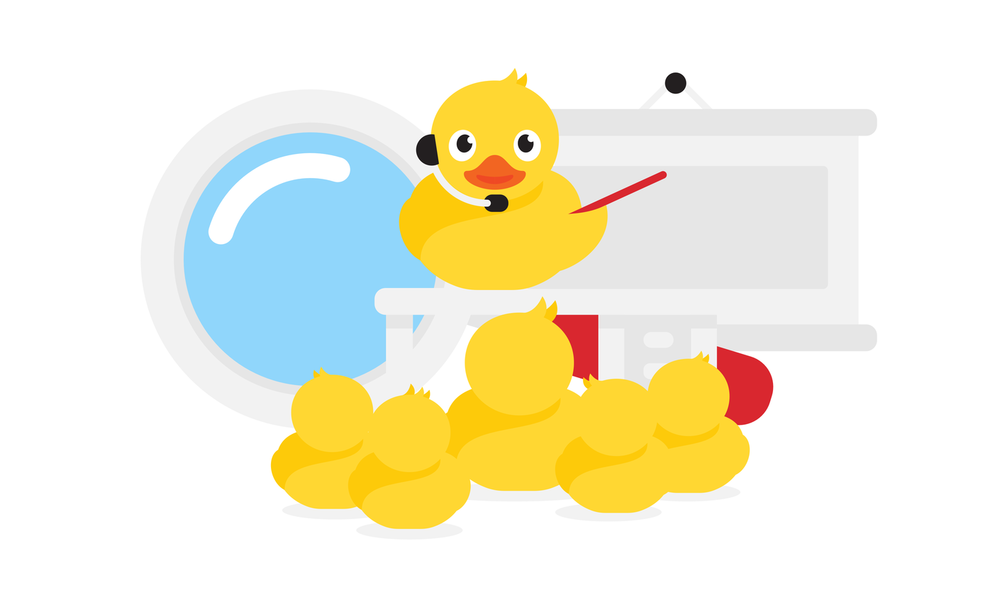
I’m sure that was a lot to take in. But the question remains – where to start? We created a project called learnQuery that will facilitate your introduction to JavaScript. You can read about it on this very same blog. I recommend going through the tasks thoroughly.
It’s critical to stay in touch with the community and keep track of successful projects, so I recommend our #FrontendCookies newsletter that brings you fresh and exciting stuff on a weekly basis.
Joining a local Frontend, JavaScript, or Web meetup is also a great idea. It will expose you to new technologies, tools, and people.
If you like podcasts take a look at JavaScript Jabber and Changelog. Additionally, Google has an excellent YouTube channel – Google Chrome developers. Finally, to get immersed in all of the latest web technologies watch Google I/O videos.
tl;dr
To recap, here are my recommendations:
- pick an editor that works for you
- start with the basics, but move up from vanilla JS to frameworks as your understanding grows
- try React once you feel you’re ready, build a simple app, and then think of adding state management if you need it
- learn and master Webpack since it’s here to stay
In attempting to write this comprehensive guide, I’m sure that I left out a bunch of important things. After you skim through all of the links, you’ll have to continue investigating on your own. I know that the price for entering this ecosystem may seem a bit steep, but the fruits it bears are sweet and plentiful. For any of you experienced JS devs out there, what recommendations would you have for people getting into JS development?