GO is a newcomer to the world of programming. An open source, statically typed programming language developed by Google that provides you with some nice features like garbage collection, memory safety and facilities for writing concurrent programs.
If you’re a developer, you’ve probably heard about it and you’re interested in a more personal opinion. Well, it’s a “C(++) like” language with an improved, simplified syntax and a few unusual features that make it stand out. It is a pretty controversial language in my opinion, taken some of its core design decisions. For example:
- You expose a public method from the module by naming it so that the first letter is uppercase
- It enforces a specific directory structure of packages by using domain names as directory names
- It doesn’t allow you to expose an undocumented public API
Upon first encounter, developers are usually horrified by this, but the philosophy behind the language is clear. To provide simple but powerful constructs with well-defined conventions, making developers more productive (and happier).
Okay, let’s make a quick overview of the GO ecosystem and dive a bit deeper.
About the language
The first thing everybody notices while learning a new language is its syntax. GO has a pretty simple and concise syntax, making it a great language to start with. You’re probably familiar with the overwhelming feeling when you’re just figuring out a new programming language. After I did “A Tour of GO” I wasn’t terrified. On the contrary, I felt like everything just made sense. Authors of the language gave their best to keep things simple.
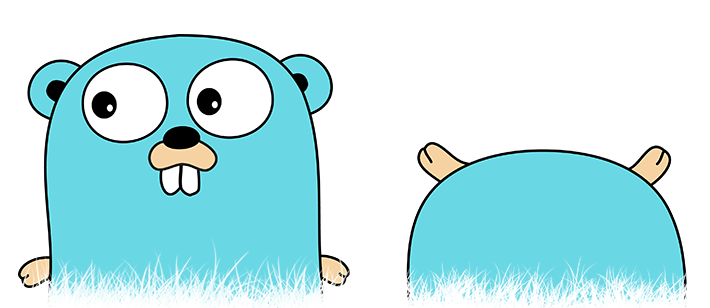
In the world of GO, everything is made out of packages which are usually designed following the main UNIX philosophy – “Do one thing and do it well”. This leads to reusable and understandable code which is a great thing, especially if you’re still learning how to program.
GO’s type system is also pretty straightforward. It revolves around structs (data objects) and methods (behavior) usually attached to those data structs. A data struct with a set of methods can satisfy an interface which is used elsewhere to achieve decoupling. This is similar to the concept of “class” most developers are familiar with, but it is done quite differently. One thing I really like about GO’s type system is that it enforces good object-oriented practices like “Composition over inheritance”. On the other hand, people starting with GO often complain that it’s missing object-oriented features and feel constrained with its approach. You’ve probably heard similar remarks made about JavaScript’s prototype inheritance.
One cool GO feature I really like is function closure. You can use it just like you would in JavaScript. Check out this Fibonacci calculator using a function closure to encapsulate the last iteration state:
package main
import "fmt"
// fibonacci returns a function that returns fibonacci numbers
// from each successive call
func fibonacci() func() int {
first, second := 0, 1
return func() int {
ret := first
first, second = second, first+second
return ret
}
}
func main() {
f := fibonacci()
for i := 0; i < 10; i++ {
fmt.Println(f())
}
}
Since GO is a statically typed language, you can expect a minimal amount of runtime errors. At Infinum, we’re mostly working with dynamic languages so runtime errors are the only errors we’re dealing with. Because of that, writing GO programs felt like a pretty relaxed job, once you get a piece of code to compile, it just works.
GO is all about concurrency, but writing concurrent programs is hard. To create a concurrent program one would usually run a thread that communicates with other threads through shared data or message passing mechanisms. GO tries to simplify this process by introducing two new concepts right into the language:
- GO routines – a lightweight thread of execution
- Channels – a connection facility between GO routines
Go ahead and try them out. It’s really simple.
GO comes with a nice set of development tools like a blazing fast compiler, code formatter, debugger, package manager etc. For example, there’s GOfmt which formats your code according to standard rules. This is not optional → you have to obey GO’s conventions. You can easily integrate GO tools with any popular text editor/IDE like Atom, Sublime, Vim or others.
Web development with GO
GO is well suited for web development. Its standard library is backed by a great net/http package providing you with everything you need to implement a simple, concurrent web server in just a few lines of code.
Here’s a simple web server example:
package main
import (
"io"
"net/http"
)
func hello(w http.ResponseWriter, r *http.Request) {
io.WriteString(w, "Hello world!")
}
func main() {
mux := http.NewServeMux()
mux.HandleFunc("/", hello)
http.ListenAndServe(":8000", mux)
}
But you probably need something more than just a standard library for your web projects, you’re probably looking for a framework.
A few popular ones are:
While some give you just basic routing support, others are trying to solve all standard problems in backend web development. In the GO ecosystem, you won’t find massive MVC frameworks (like Ruby on Rails) that solve just about everything, since that doesn’t fit well with the core philosophy of the language. You’ll find simpler and more focused packages because Gophers believe that’s the way software should be built.
While developing backend apps, you usually need a data persistence layer of some sort. A quick Google search will give you something like:
- gorm – full ORM library
- gorp – simpler persistence layer
- sqlx – a low-level extension to GO’s standard database/sql package
And what about view rendering? Well, there’s a cool html/template package giving you basic building blocks for connecting HTML templates with your program, or encoding/json if you’re building an API and need to render some JSONs. If you want a complete view renderer that abstracts a few things, you can check out the render package that plays nicely with many middleware libraries mentioned above.
So, if you want to do web development in GO you can find a package for almost everything. Here’s a long list of libraries that might prove useful. Be cautious, this is a pretty young ecosystem and you could encounter a lack of documentation or support while using some of those packages.
Personal experience
The idea was to create a web app that’s simple, useful and covers standard problems in web development (routing, database, connecting to other APIs). The result was an app called “Managing dependency management”. It scans public GitHub repositories, analyses root package.json files and prints out which dependencies (npm packages) are out of date. We used martini web framework, postgres database, gorm data layer, GitHub’s library and managed to make everything work in just under 8 hours.
GO in action
After our test run proved to be successful, we decided to incorporate GO in our client work. We’re working with Startup Threads, a US company that prints and sends custom T-shirts worldwide.
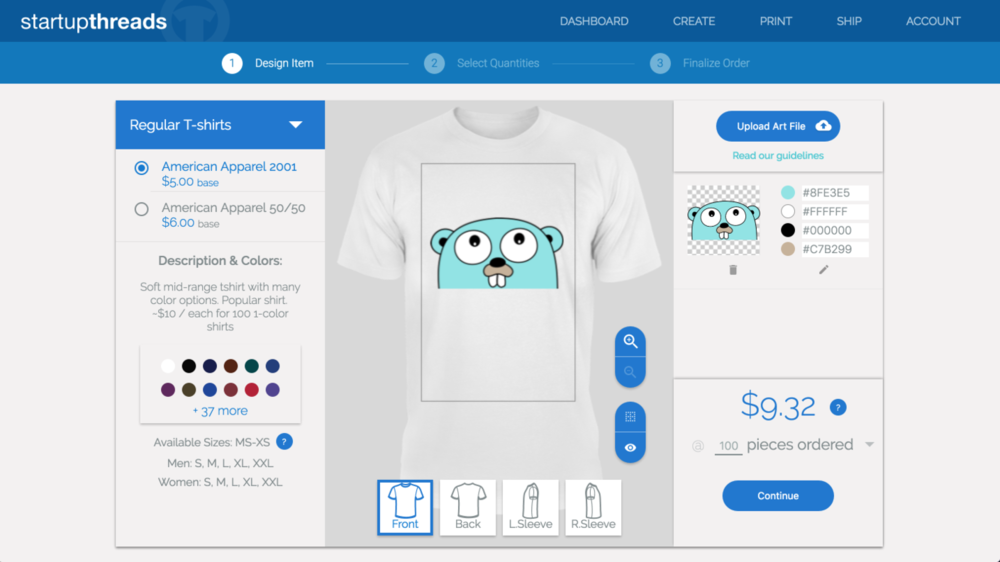
We’ve built a fast web server in GO that does image analysis on user-uploaded print art. It’s a scalable, Heroku-based architecture which was fairly easy to setup. In the end, GO made automatic deployment really fast and reliable.
Wait! But why GO?
You’re probably asking yourself this question. Why should I use GO when there are other, more established and mature ecosystems for web development? GO’s main selling point is its right combination of productivity, performance and built-in concurrency support. So, if you’re building a simple CMS-like website, GO is not the best tool for the job, but if you need in order to build a high-performance server that handles thousands of requests per second, then GO might be the right fit.
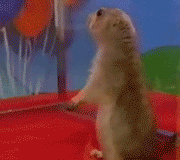
Playing with GO was a great experience and I’ll definitely try building more complex systems with it. The most important benefit is the productivity and easy collaboration achieved through its simplicity and well-defined conventions. Of course, it is not meant to be used for everything, so before you decide to use GO for your next project, research whether it is the right tool for the job.
Have a cool GO project you need help on? Contact us.
Want to know more?
Check out these useful links: