With automation tools like Playwright, testing web accessibility doesn’t have to be tedious or time-consuming. Learn how to speed up your accessibility checks using Playwright and axe-core, and simplify the path to building more inclusive digital products.
Ensuring your web page is accessible to all users is becoming increasingly important for businesses. With regulatory pressures mounting and public awareness rising, brands and companies are looking to optimize their digital products to meet accessibility standards.
However, testing a web page for accessibility is a nuanced task, and certain aspects of it can be particularly time-consuming. Fortunately, there are a number of tools available to help testers check web accessibility thoroughly and efficiently, especially by leveraging test automation.
Not every team has the resources or bandwidth to build custom tools, but existing solutions can already achieve a great deal. In this article, we’ll explain how to conduct accessibility testing using Playwright, a very popular tool in the testing community. With just a few simple adjustments, Playwright can significantly speed up and enhance the efficiency of your testing process.
Why digital accessibility is important
A significant portion of the population faces challenges when accessing digital resources due to physical, cognitive, and sensory impairments. According to the World Health Organization, approximately 16% of the global population experiences significant disabilities.
If your app or website isn’t built with accessibility in mind, you risk excluding a considerable number of users – not only those with permanent disabilities but also individuals experiencing temporary or situational impairments, such as a broken arm or navigating a digital product in a noisy environment. Accessibility standards ultimately improve the user experience for everyone.
Beyond ethical considerations, accessibility is increasingly becoming a legal necessity. In the United States, the Americans with Disabilities Act mandates that public accommodations, including websites, must be accessible to individuals with disabilities. Similarly, the European Union’s European Accessibility Act and the Web Accessibility Directive establish clear guidelines for digital accessibility. Non-compliance can lead to significant legal consequences, including lawsuits, fines, and reputational harm.
What is accessibility testing?
Accessibility testing is the process of identifying barriers that may prevent users from fully interacting with a website or service. It ensures that people with disabilities can access and navigate the content just as easily as anyone else.
The testing is usually conducted through a combination of automated and manual accessibility checks. It evaluates several aspects, including visual design, interactive elements, content structure, navigation, and multimedia components.
Can accessibility testing be automated?
Accessibility testing can be automated to a certain extent. Automation tools can help us identify many common accessibility issues and provide reports that highlight areas needing improvement. These tools can check for issues like missing alt text, poor color contrast, improper heading structures, and missing ARIA attributes – areas frequently overlooked during development.
However, automated tools are limited to verifying basic accessibility compliance and cannot evaluate how intuitive or user-friendly an interface is. Human testing remains vital for uncovering issues such as confusing navigation, unintuitive layouts, or frustrating interactions, which are all essential if we want to ensure a truly inclusive user experience.
Automating accessibility testing with Plawright and axe-core
Playwright is an excellent starting point for teams interested in accessibility test automation. It is a widely used, open-source framework known for its ease of setup and strong community support. More importantly, it offers integration with axe-core, a powerful accessibility testing library created by Deque Systems. Axe-core helps testers efficiently detect violations of accessibility standards, such as the Web Content Accessibility Guidelines (WCAG), and provides insights on fixing any issues found.
Playwright and axe-core are a powerful combo – one that helps testers streamline accessibility checks, improving both efficiency and accuracy in their testing processes.
Initial setup
We’ll start by installing Playwright using npm
.
npm init playwright@latest
You’ll be prompted to answer a few setup questions – feel free to go with the default options. TypeScript should be selected by default, but double-check that it is.
For full installation details, check the official Playwright documentation.
Next, add the additional packages needed for this implementation:
npm install @axe-core/playwright
npm install axe-html-reporter
Then, import the necessary modules in your test file:
import { test, expect } from '@playwright/test';
import AxeBuilder from '@axe-core/playwright';
import { createHtmlReport } from 'axe-html-reporter';
Page inspection
Now, let’s walk through a practical example using todoMVC. To inspect a page for accessibility issues, we’ll use AxeBuilder
from the axe-core/playwright package
. The command new AxeBuilder({ page }).analyze()
tells Axe to scan the current page and identify any violations according to established standards, and the results are stored in the accessibilityScanResults
variable.
const accessibilityScanResults = await new AxeBuilder({ page }).analyze();
To focus the analysis on specific standards, you can refine the accessibility scan by using the withTags()
method. For example, if you’re targeting the most common WCAG compliance levels (WCAG 2.0 and WCAG 2.1, levels A and AA), you can do so as follows:
const accessibilityScanResults = await new AxeBuilder({ page })
.withTags(['wcag2a', 'wcag2aa', 'wcag21a', 'wcag21aa'])
.analyze();
Assertion
After performing the scan, we need to verify whether any accessibility violations have occurred. You can achieve this by asserting that the violations array within the results is empty. If any violations are found, your test will fail, prompting you to address the identified issues:
expect(accessibilityScanResults.violations).toEqual([]);
Creating the report
To generate a readable summary of the accessibility scan, we’ll use the createHtmlReport
function from the axe-html-reporter
package. This function takes in the scan results we previously stored in accessibilityScanResults
. We can also use options to configure the report name and output directory.
const reportHTML = createHtmlReport({
results: accessibilityScanResults,
options: {
outputDir: reportDir,
reportFileName: reportName,
},
});
Note that createHtmlReport
returns an HTML string – it doesn’t create a file by itself. To write the report to disk, we’ll use Node’s fs
module:
if (!fs.existsSync(`${reportDir}${reportName}`)) {
fs.mkdirSync(`${reportDir}${reportName}`, { recursive: true });
}
fs.writeFileSync(`${reportDir}${reportName}`, reportHTML);
Parametrizing the test
Testing a single page is a good start, but broader coverage requires more. Let’s modify our test to run against multiple pages by creating an array of objects containing URL/name
pairs. The URL
field tells Playwright which page to check, and the name
field isn’t required, but helps us generate more descriptive report names:
const urlsToCheck = [
{
url: 'https://todomvc.com/examples/react/dist/',
name: 'reactPage',
},
{
url: 'https://todomvc.com/examples/angular/dist/browser/#/all',
name: 'angularPage',
},
{
url: 'https://todomvc.com/examples/javascript-es6/dist/',
name: 'jsPage',
},
];
Next, we’ll loop through each URL in our test using forEach
:
test.describe('Accessibility', () => {
urlsToCheck.forEach(({ url, name }) => {
test(`should check: ${url}`, async ({ page }, testInfo) => {
…
This setup generates a separate, clearly labeled report for each tested page.
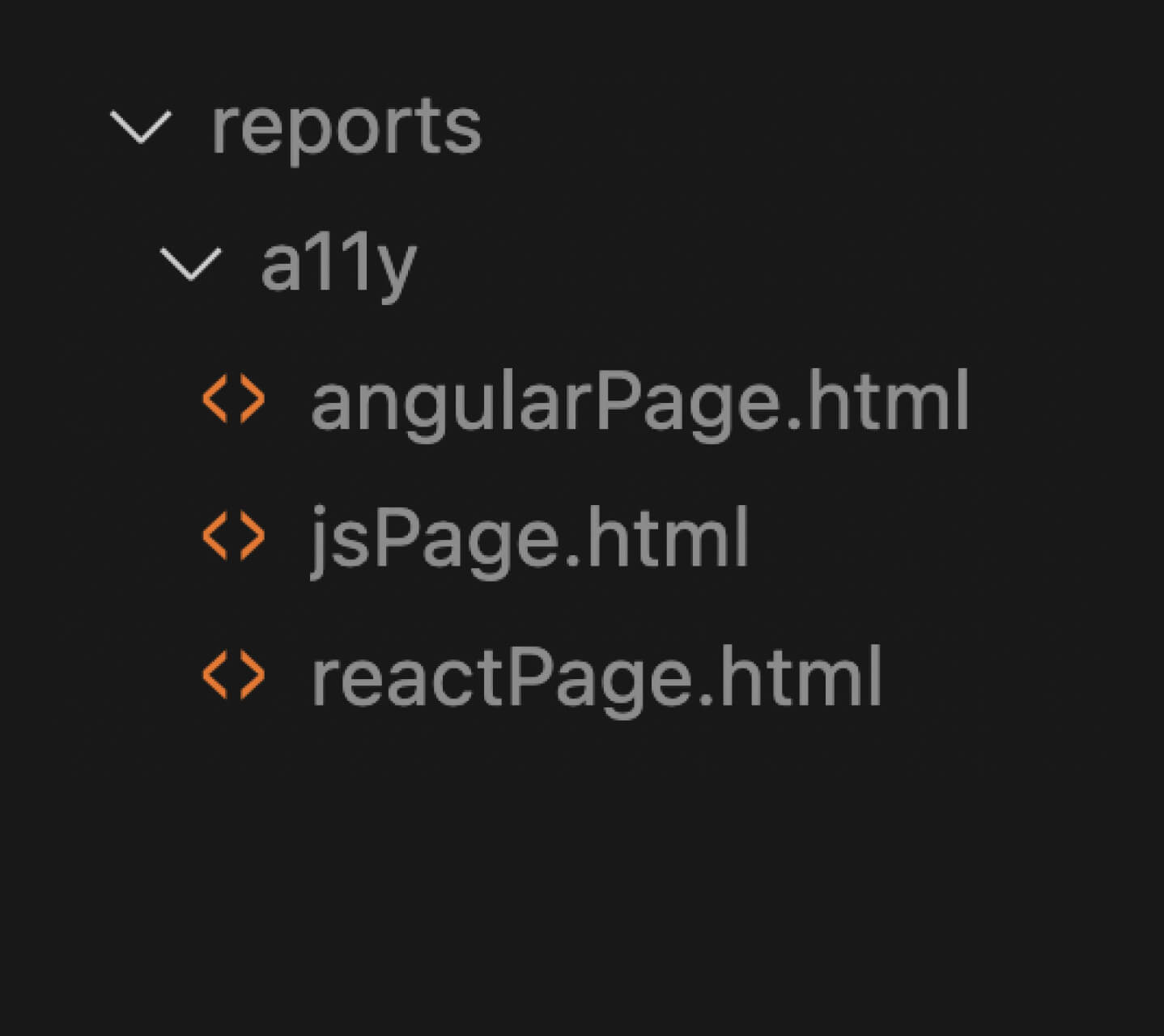
The full example
Here’s how the complete test script comes together. This is a simple example that shows how easy it is to get started with accessibility test automation using Playwright and axe-core:
import { test, expect } from '@playwright/test';
import AxeBuilder from '@axe-core/playwright';
import { createHtmlReport } from 'axe-html-reporter';
import fs from 'fs';
const reportDir = 'reports/a11y/';
const urlsToCheck = [
{
url: 'https://todomvc.com/examples/react/dist/',
name: 'reactPage',
},
{
url: 'https://todomvc.com/examples/angular/dist/browser/#/all',
name: 'angularPage',
},
{
url: 'https://todomvc.com/examples/javascript-es6/dist/',
name: 'jsPage',
},
];
test.describe('Accessibility', () => {
urlsToCheck.forEach(({ url, name }) => {
test(`should check: ${url}`, async ({ page }, testInfo) => {
const reportName = `${name}.html`;
await page.goto(url);
// Scan the page and save results to accessibilityScanResults
const accessibilityScanResults = await new AxeBuilder({ page }).analyze();
// Create HTML report
const reportHTML = createHtmlReport({
results: accessibilityScanResults,
options: {
outputDir: reportDir,
reportFileName: reportName,
},
});
// Save HTML report to a file
if (!fs.existsSync(`${reportDir}${reportName}`)) {
fs.mkdirSync(`${reportDir}${reportName}`, { recursive: true });
}
fs.writeFileSync(`${reportDir}${reportName}`, reportHTML);
// Assert no violations detected
expect(accessibilityScanResults.violations).toEqual([]);
});
});
});
Report
The reports generated by axe-core are clear, detailed, and practical. They highlight each accessibility violation, categorize its severity, and point to the relevant WCAG guideline. For every issue, you’ll see the specific DOM element affected and get actionable suggestions on how to fix it.
What’s especially helpful is that each issue comes with documentation links, allowing testers and developers to learn more about the problem and why it matters – making it easier to address issues effectively and build more inclusive products.
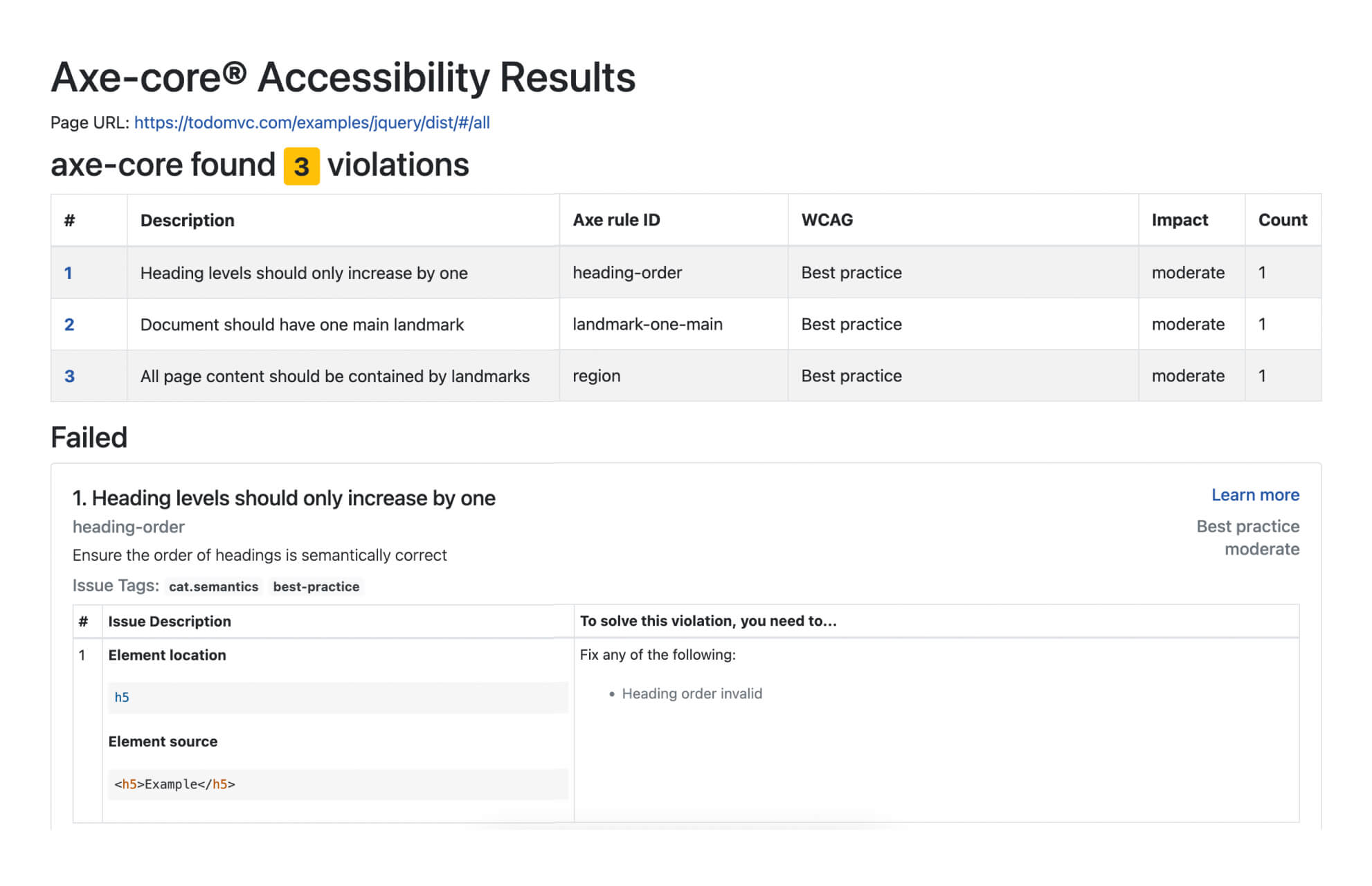
Make accessibility testing work for you
Digital accessibility is no longer a nice-to-have – it’s becoming increasingly relevant for businesses looking to provide equal access for all users and reduce legal and reputational risks. However, evaluating accessibility manually can be time-consuming and prone to human error. Test automation can be a great asset for testers, and tools like Playwright and axe-core make it easy to catch many common accessibility issues early in the development process.
As our example shows, setting up automated tests doesn’t require complex custom solutions – just a few lines of code and the right tools. Still, we need to bear in mind that automation isn’t a silver bullet. Automated tests are powerful allies, not replacements for human judgment. Experienced testers use these tools to boost efficiency and consistency, while still applying manual testing to assess usability, context, and the overall user experience.
Ultimately, the best approach combines speed with empathy – automating what you can and thoughtfully testing what you can’t.