Rails vs. Django, an age-old debate in the web-development community. In this article, I’ll try to cover my own experience of moving from a Python-based web framework to a Ruby-based one.
The story begins with me graduating from university. My first job was in a media company that runs a number of large web portals, news websites and other online publications. I worked on developing and maintaining those websites as a Django developer. As you can imagine, it was a great and challenging job for a fresh programmer. But just as I settled in and became comfortable working in Django, an opportunity arose to work at Infinum as a Ruby on Rails developer.
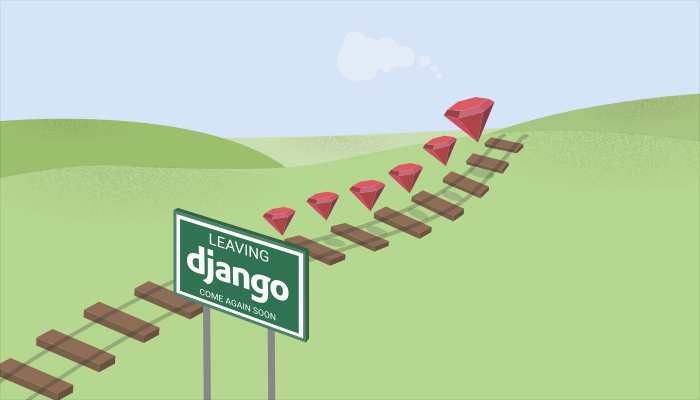
Getting started is weird
It was a tough decision for me, but I decided to be brave and accept new challenges. One of the most motivating things for me was the fantastic work atmosphere and culture here at Infinum. I decided to learn Rails, and now, after 4 months, I have no regrets about making the switch.
In the beginning, some concepts were a bit strange to me. The Rails “convention over configuration” philosophy caused some confusion in my head because there was too much “magic” going on. When people talk about “magic” in Rails, they usually refer to things that the framework does “on its own”, but which you are required to know about. This is described in this StackOverflow article:
Well, consider a couple bits of Rails “magic”: when you write a controller class, its methods have access to certain variables and certain other classes. But these variables and classes were neither defined nor imported by anything in the file of Ruby code you’re looking at; Rails has done a lot of work behind the scenes to ensure they’ll just be there automatically.
And when you return something from a controller method, Rails makes sure the result is passed along to the appropriate template; you don’t have to write any code to tell it which template to use, where to find it, etc., etc.
In other words, it’s as if these things happen by “magic”; you don’t have to lift a finger, they just happen for you.
I had to learn to be more careful about naming conventions and the directory structure because Rails relies on those things to work its magic.
Shortly after that, I started noticing many similarities to the Django framework and very soon I learned how to do things in Rails that I knew how to do in Django. Almost everything that’s possible to do with one framework is doable in the other as well. The time required to start working on real-world projects was significantly shorter because of my Django experience.
Writing more tests
What confused me at first later became the thing that I love about Rails. Rails’ magic results in higher productivity and a cleaner code, for example in controllers. Controllers can look really nice, short and sweet. I like the Rails RESTful orientation – routes and controllers are designed in a way that it is best for you to follow the REST architecture.
# in routes.rb
resources :orders, only: [:index, :show, :new, :create]
# orders_controller.rb
class OrdersController < ApplicationController
def index
@orders = Order.where(active: true)
end
def show
@order = Order.find(params[:id])
end
def new
@order = Order.new
end
def create
@order = Order.new(params[:order])
if @order.save
redirect_to @order
else
render ’new’
end
end
end
At Infinum, I started to write more tests than before. Rails places extra emphasis on testing and soon I realized how useful tests can be. Many great gems are made in order to make testing simple and clean.
The Rails asset pipeline is a great solution for managing static files (CSS, JS). I like the fact that I don’t need to worry about concatenating and minifying them. In Django, there is no built-in solution for this problem.
What I miss from Django
There are some things from Django I still miss. For example, I miss Django’s way of database migration. When you want to make a change to some table, all you have to do is directly change the model file and start the migration. When you change the model, the corresponding table in the database also changes. This way, you have your model definition and fields in one place.
In contrast, in Rails you don’t change the model file, but generate a migration file for every change you want to make. You don’t have a list of model fields in the model definition and you cannot change it directly like you can in Django.
Here is an example of how you do database migration in Django:
# Author model before migration
class Author(models.Model):
name = models.CharField(max_length=80)
slug = models.SlugField(max_length=80)
is_active = models.BooleanField(default=True)
# We want to add new field called image
class Author(models.Model):
name = models.CharField(max_length=80)
slug = models.SlugField(max_length=80)
# we add image field on third place
image = models.ForeignKey(Image, null=True, blank=True)
is_active = models.BooleanField(default=True)
# Now we only have to start the migration from the command line
$ python manage.py makemigrations
$ python manage.py migrate
Another thing I miss is Django’s great admin interface that you get out of the box when you create a Django project and database tables. It’s of great help when you want to view or change some data.
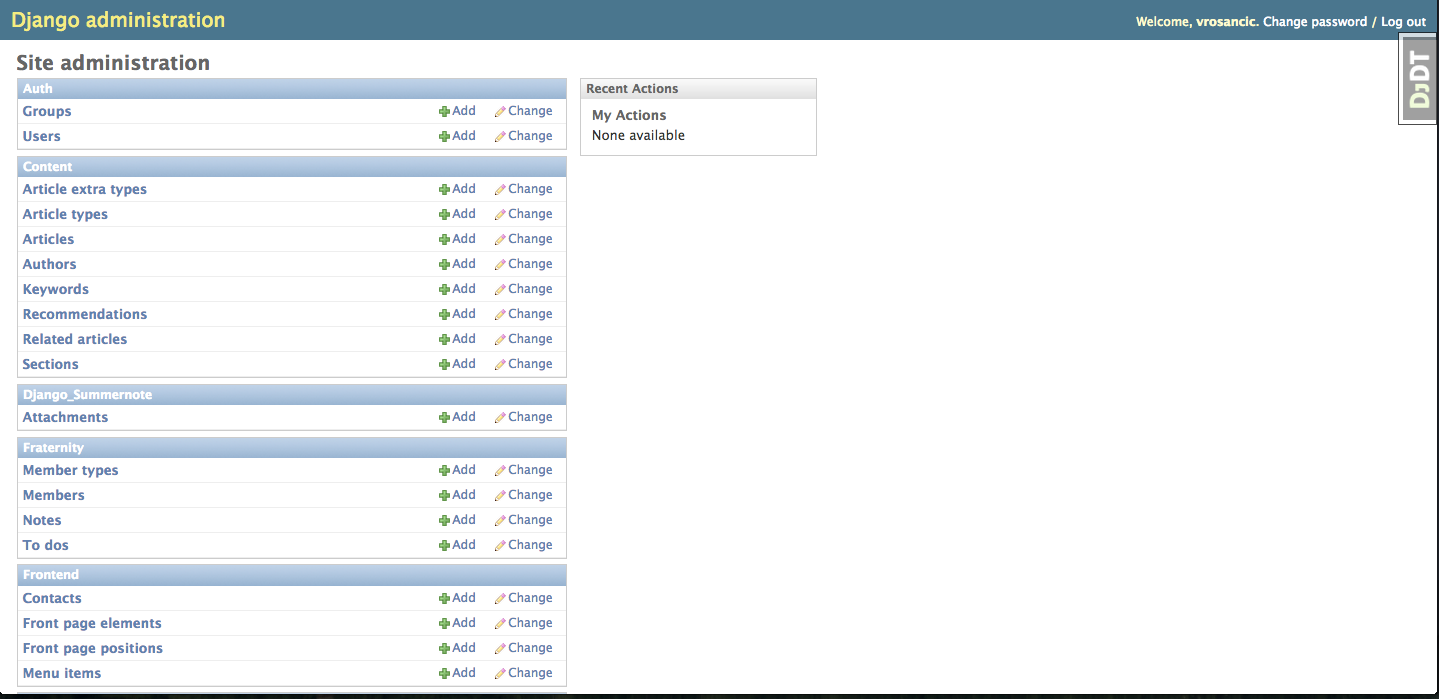
Last but not least, I like Django’s structure based on apps. Every app is responsible for a specific behavior and segment of a larger system. Apps have their own models, views and templates and they can be connected to other apps. This way, you get cleaner structure when you have a big application and a lot of files and directories.
Conclusion
All things considered, both frameworks are really good and both of them are making the process of building modern web applications simpler. If you are willing to put in some time and effort, it’s not hard to switch from one to another.
Here’s some advice – try to focus on the upsides of the target framework. I got used to the Rails way of thinking and now I’m really enjoying the platform’s signature behavior – the ability to write very concise and clean yet very powerful code.
As I said in the beginning, I moved from one good framework to another, and nothing terrible happened. Try it yourself – it’s easier than you think.