Back in 2015, we published an article discussing the top five Android libraries we used at the time. However, the Android platform has grown rapidly since then. As you know, in the fast-paced field of software development, things change and evolve at lightning speed. That’s why we’ve decided it’s time for an updated list of the ultimate Android libraries.
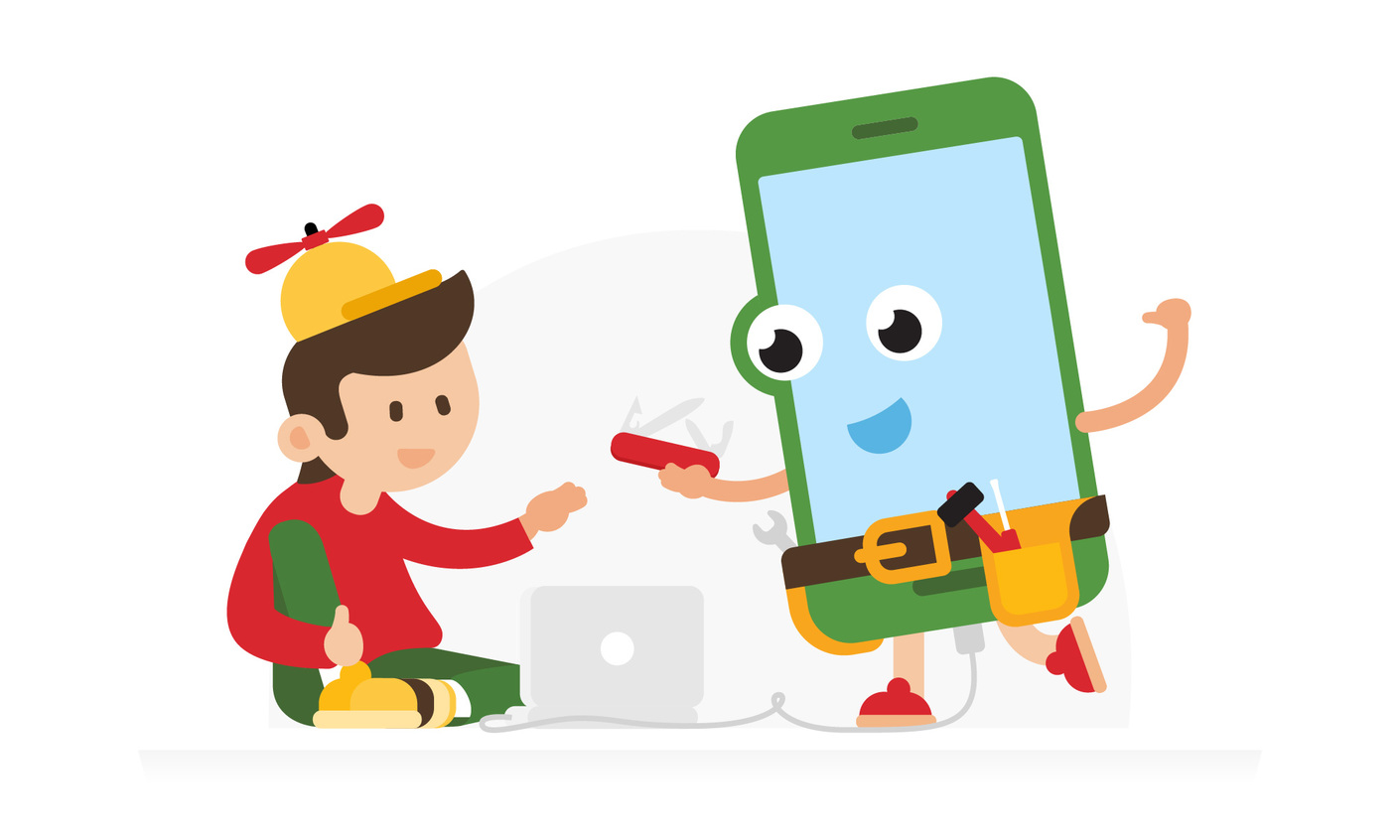
Without further ado, let’s jump right into our list. We’ll kick off with one of the oldest, and I’d argue, one of the most useful Android libraries.
1. Retrofit
Retrofit is type-safe HTTP client that allows you to define your REST API as an interface. You can manipulate the API requests’ body, headers, query parameters, and much more via annotations, which makes everything clean and simple. Retrofit also allows synchronous and asynchronous API calls execution.
interface ApiService {
@GET("movie/{id}")
fun getMovieDetails(@Path("id") id: String) : Call<MovieDetails>
}
To top it off, Retrofit offers a separate Rx module. If you are using Rx, this module returns your API call as an Observable so you are able to chain it with the rest of your application. These are just a few of the many reasons we at Infinum still love and use Retrofit.
2. Moshi
Moshi is a library that converts JSON into Java and Kotlin models. A lot of people refer to the Moshi as GSON 3.0. This library is superior to GSON in several aspects: [it’s faster](https://stackoverflow.com/a/43578738/3920456 “StackOverflow”), it includes Kotlin support, it’s maintained, it throws predictable exceptions, and it doesn’t use broken DateTime
adapter by default. Plus, converting JSON to Java model (and vice-versa) is straightforward with Moshi.
val moshi = Moshi.Builder().build()
val jsonAdapter = moshi.adapter(Model::class.java)
/* JSON to Model */
val model = jsonAdapter.fromJson(json)
/* Model to JSON */
val json = jsonAdapter.toJson(model)
We also admire Moshi for its JSON API support. JSON API is the specification for building APIs, and a lot of APIs we work with are written using that specification. Moshi JSON API makes our life easier because it converts JSON API responses into meaningful Java objects. Retrofit also has support for Moshi, and it all just clicks together.
3. Chuck
Chuck is an HTTP inspector for Android that allows you to dig into your application’s HTTP history on your mobile phone. The HTTP log is displayed as a notification, which you can expand to open full Chuck UI. When you use Chuck, your quality assurance team will applaud you as they will be able to see whether an issue persists on Android or the backend side. This library is also sometimes more useful than logcat. That’s because your HTTP history persists even if the app is killed, while logcat sometimes cleans itself after the app is restarted.
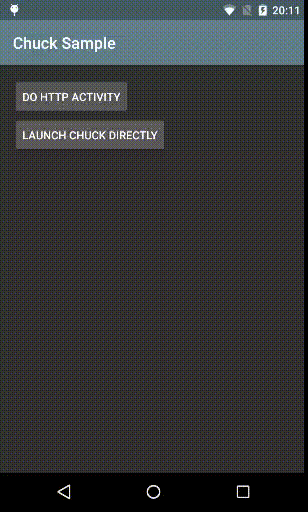
4. Glide
As you probably know by now, Android image loading and handling API is terrible. It’s a nightmare to even resize an image without getting “OutOfMemoryException.” Glide is an image loading library that exposes a nice API, allowing you to transform your image however you want.
GlideApp.with(context)
.load(url)
.centerCrop()
.placeholder(R.drawable.ic_placeholder)
.into(imageView)
This library allows you to easily load a remote image into your ImageView, define fallback images, cache and scale your images, and much more. Try accomplishing all this without Glide, and you will quickly understand why this has become our core library. It even supports some common transformations like a circular image out of the box.
5. ThreeTen
ThreeTen is a date and time handling library for Android. It is a backport of JSR-310, which was included in Java 8 as a standard “java.time.*” package. We love this library because the standard Android Calendar API is a nightmare to work with.
/* Current DateTime */
LocalDateTime.now()
/* String to DateTime */
val localDateTimeString = "2011-12-03T10:15:30"
val localDateTime = LocalDateTime.parse(localDateTimeString)
ThreeTen is much smaller than JodaTime in terms of method count and binary size. Its API is also more concise than JodaTime’s API. If you are currently using JodaTime, it is probably not worth the switch to ThreeTen. However, if you are starting a new project, I strongly suggest you try out ThreeTen instead.
6. Timber
Timber is powerful, yet simple, logging library built on top of Android “Log” class. It allows you to easily turn logging on or off. It also offers nice support for logging formatted strings and exceptions. Because of all these benefits, Timber is one of the core libraries we use on almost all our Android projects.
/* Log error */
Timber.e("Error happened")
/* Log warning with params */
Timber.w("Your variable value is %s", variable)
/* Log exception */
try {
...
} catch (e: Exception) {
Timber.e(e)
}
7. Room
Room is an official Android ORM, and there are multiple reasons for that status. This library features a beautiful API that is similar to Retrofit. It also relies heavily on annotations and standard SQL syntax.
@Dao
interface MovieDao {
@Query("SELECT details FROM movies WHERE id = :id")
fun getMovieDetails(id: String): MovieDetails
}
Additionally, Room includes out-of-the-box support for Rx and “LiveData,” so you can decide to use it however you like. The main benefit Room offers over other ORMs is its simplicity. Other ORMs come with much more complex APIs that require you to read all the documentation thoroughly before you can use them. Thanks to its standard SQL syntax, Room is incredibly easy to understand, allowing you to dive in without spending hours reading the documentation.
8. RxJava
RxJava is a Java implementation of ReactiveX API that allows you to chain asynchronous tasks and events into observable sequences. Users expect modern applications to display data in real-time. In other words, they want to see data updated automatically. That’s where RxJava can help.
When fetching real-time data, it becomes extremely difficult to merge multiple API calls, switch threads, and handle errors. This is where RxJava shines, and it is the reason why we started using this library. I will admit that RxJava is confusing and hard to learn, but it is completely worth your time. Even after we switched to Kotlin, we continued to use RxJava because of its benefits. Together with Kotlin, the API is even better thanks to the additional extension functions.
Single.zip(
/* Execute first API call and retry twice if it fails */
apiService.getMovieActors(id).retry(2),
/* Execute second API call and retry twice if it fails */
apiService.getMovieDetails(id).retry(2),
/* Receive successful results and merge them into single model */
BiFunction<List<Actor>, MovieDetails, Movie> { actors, details -> Movie(details, actors) }
)
/* Execute API calls on IO thread */
.subscribeOn(Schedulers.io())
/* Receive results on MainThread */
.observeOn(AndroidSchedulers.mainThread())
.subscribeBy(
onError = { /* Handle error */ },
onSuccess = { /* Handle full movie data */ }
)
Try accomplishing something similar to the above with plain Java. I dare you.
9. Android KTX
Android KTX is a set of Kotlin extensions that wraps Android API, making it more user-friendly. The whole purpose of this library is to make Android API more pleasant to use. It adds a lot of methods and cool new features of Kotlin, such as named parameters, lambdas, and default parameter values.
/* Display View */
view.isVisible = true
/* Apply padding to all sides */
view.setPadding(padding)
/* Update padding on any side */
view.updatePadding(left = newPadding, right = newPadding)
/* Quick Toast */
toast("Display test toast")
/* New way to create bundle */
bundleOf {
"key1" to item1
"key2" to item2
}
/* Better way to use SharedPreferences */
sharedPreferences.edit {
putString("key1", "value1")
putString("key2", "value2")
}
Android KTX features a lot of extension functions, and the only way to learn about them is by digging through library code to see how they improved Android API. This library changes the way we consume Android API—and for that reason, it should become the core of every Android application written in Kotlin.
10. Dagger
Without Dagger, our Top 10 libraries list would be incomplete. Dagger is a fully static, compile-time dependency injection framework. Similar to RxJava, Dagger is really hard to understand (it took me a while to understand their CoffeeMaker example) but completely worth your time and effort.
Dependency injection is a way to provide smaller components to another model and glue them together with minimum effort. For example, if you have a Car model, you can provide tires to it and easily replace the tire implementation in the future—all without having to change a single line in your Car model.
When developing larger applications, you don’t want to handle dependency injection yourself because the code will grow quickly and become extremely difficult to maintain. Dagger helps you avoid this because it creates your dependency injection graph in compile-time via annotation processing.
In the last year, Google also included a separate Dagger Android module, which allows you to write less boilerplate code and inject dependencies easier.
Conclusion
So, there you have it: Our 2018 List of Top 10 Android Libraries. These ten superior options make Android development much more enjoyable for anyone who uses them. Looking for more options? It just so happens that we at Infinum have developed some Android libraries of our own. Check them out:
- MjolnirRecyclerView – extends RecyclerView with support for headers, footers, empty view, and DiffUtils
- Goldfinger – simplifies Fingerprint authentication implementation
- DBInspector – view and share your app databases
- Complexify – an easy way to check the quality of a user’s password
- Prince of Versions – handle app updates inside the app
If you want to hear about newest Android libraries, subscribe to our weekly Android newsletter